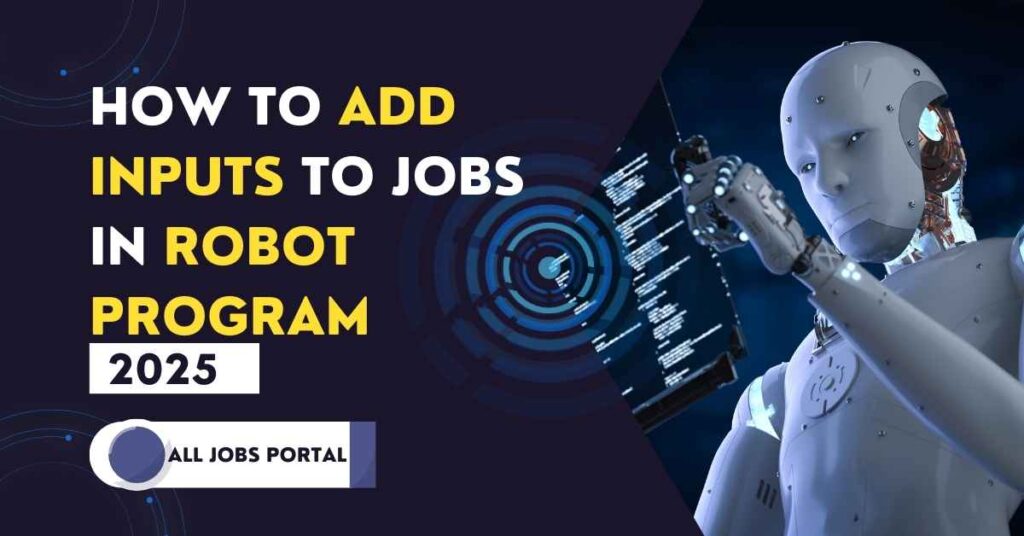
Robot programming is the process of creating and inputting instructions that enable robots to perform specific tasks autonomously. Adding inputs to jobs in robotics is a critical aspect of programming, as it allows robots to adapt to varying tasks, environmental changes, and operational requirements. This flexibility ensures that robots can handle complex workflows with precision and consistency.
Incorporating inputs enhances automation by enabling real-time decision-making, reducing downtime, and increasing productivity. It also optimizes efficiency by minimizing human intervention, resulting in cost savings and improved accuracy. Mastering this process is essential for industries leveraging robotics to stay competitive in a rapidly evolving landscape.
Understanding Inputs in Robot Programs
In robot programming, inputs are signals or data received by the robot from various sources, enabling it to perform tasks, make decisions, and interact with its environment. These inputs are critical for ensuring flexibility and adaptability in robotic operations, whether in industrial automation, healthcare, or research applications.
What are Inputs in Robot Programming?
Inputs serve as the communication bridge between the robot and its surroundings. They provide the information needed for the robot to understand and respond appropriately to its environment. By integrating inputs, programmers can equip robots with the ability to make real-time decisions, ensuring precision and efficiency in tasks.
Examples of Inputs
Inputs in robot programming come from diverse sources. Here are some examples:
- Sensors:
- Proximity sensors for detecting nearby objects.
- Temperature sensors for monitoring environmental conditions.
- Cameras or vision sensors for image recognition and analysis.
- User Commands:
- Manual instructions entered via a control panel or software interface.
- Voice commands or touch inputs for user-friendly operation.
- External Devices:
- Signals from other machinery in an integrated system.
- Data from IoT (Internet of Things) devices for synchronized operations.
How Inputs Impact Robot Performance
The role of inputs in robot programming extends beyond mere data collection. They directly influence the robot’s performance, enabling:
- Adaptability: Robots can adjust their behavior based on dynamic inputs, such as changing object locations or varying environmental conditions.
- Precision: By using real-time feedback, robots can refine their actions, reducing errors and ensuring accuracy.
- Efficiency: Automating responses to inputs reduces the need for manual intervention, speeding up operations and increasing throughput.
Step-by-Step Guide: Adding Inputs to Robot Programs
Integrating inputs into a robot program involves systematic steps to ensure that the robot processes the information correctly and responds as intended. Below is a detailed guide:
Step 1: Identify the Type of Input Required
- Determine the purpose of the input.
- Example: Use a proximity sensor to avoid collisions or a camera for object detection.
- Match the input type to the task at hand:
- Physical inputs like sensors for environmental interaction.
- Logical inputs like user commands for task-specific control.
Step 2: Access the Robot Programming Environment
- Open the software or interface used for programming the robot.
- Examples: ABB RobotStudio, FANUC Roboguide, or Universal Robots’ Polyscope.
- Ensure the programming environment is compatible with the input hardware.
Step 3: Define Input Variables
- Create variables within the program to represent the inputs.
- Example: Define a variable named sensor_signal for input from a proximity sensor.
- Specify the data type for each variable (e.g., Boolean, integer, or string).
- Boolean inputs can indicate on/off states, while numerical inputs can represent specific measurements.
Step 4: Map Inputs to Specific Actions
- Assign logical operations to each input.
- Example:
- If the sensor_signal is triggered, the robot stops moving.
- If the user_command is “start,” the robot begins the assigned task.
- Example:
- Use conditional statements to implement these actions in the program.
Example in pseudocode:
IF sensor_signal = TRUE THEN
Robot.Stop()
ELSE
Robot.MoveForward()
ENDIF
Step 5: Test the Integration
- Simulate the Program: Use a virtual environment to test the input functionality before deploying it to the physical robot.
- Perform Real-World Testing:
- Connect the input devices to the robot.
- Observe how the robot responds to different input conditions.
- Debug as Needed: Identify and resolve any errors to ensure seamless operation.
Cruise Ship Jobs in Dubai: Complete Guide For 2025
Benefits of Adding Inputs to Robot Programs
Incorporating inputs into robot programs yields several benefits:
- Enhanced Safety: Inputs like proximity sensors help prevent accidents by stopping the robot when an obstacle is detected.
- Improved Flexibility: Robots can handle diverse tasks by responding to varying inputs.
- Cost Savings: Automation based on real-time data minimizes manual intervention, reducing labor costs.
- Better Productivity: Inputs allow robots to operate continuously and efficiently, boosting output.
Popular Techniques for Adding Inputs
Integrating inputs effectively into robot programs is essential for ensuring their adaptability, precision, and efficiency. Below are some widely used techniques to add and utilize inputs in robotic programming:
1. Using IF Conditions in CALL Statements
One common approach is leveraging conditional statements like IF to trigger specific actions or subroutines based on the input’s status. This technique enables real-time decision-making by calling particular sections of the program when input conditions are met.
Example:
If a proximity sensor detects an object (input_signal = TRUE), the robot stops and executes a predefined action.
IF input_signal = TRUE THEN
CALL StopRoutine
ELSE
CALL ContinueRoutine
ENDIF
Benefits:
- Simplifies programming by dividing tasks into manageable routines.
- Increases flexibility, as robots can adapt to changing conditions dynamically.
2. Integrating Inputs via Sensors and PLC Systems
Sensors and Programmable Logic Controllers (PLCs) are widely used for input integration in industrial settings.
- Sensors: Provide real-time data such as proximity, temperature, or vision-based inputs, helping robots interact with their surroundings.
- Example: A light sensor triggers a robot to pick up an object only when the object is in position.
- PLCs: Act as intermediaries between the robot and external devices, collecting and transmitting inputs to the robot program.
- Example: A conveyor belt’s PLC sends signals to the robot, indicating when to load or unload items.
3. Writing Logic for Decision-Making Based on Input Status
Robotic programs often include logical operations to decide the next course of action based on input conditions. Decision-making logic typically involves:
- Conditional Statements: Used to trigger specific actions.
- Loops: Employed to repeatedly check inputs and respond accordingly.
Example in Pseudocode:
vbnet
Copy code
WHILE sensor_input = FALSE DO
Robot.Wait()
ENDWHILE
Robot.PickObject()
This code ensures that the robot waits until a sensor detects an object before performing the next task.
How to Get a Job in Qatar 2025: Complete Guide
Best Practices for Input Integration
To maximize efficiency and reliability, it is crucial to follow best practices during input integration:
1. Ensuring Input Reliability and Accuracy
Accurate and reliable inputs are fundamental for consistent robot performance.
- Calibrate Sensors Regularly: Ensure that sensors provide precise data by conducting regular calibration.
- Use Shielded Cables: Prevent electrical interference that may distort input signals.
- Filter Noisy Signals: Implement signal filtering techniques to eliminate unwanted fluctuations in sensor data.
2. Testing and Debugging the Robot Program
Thorough testing and debugging help identify and resolve issues in the program before deploying it in real-world scenarios.
- Simulate the Program: Use simulation software to test input functionality and identify potential errors.
- Conduct Real-World Trials: Test the program in controlled environments to evaluate its behavior under actual operating conditions.
- Debugging Tools: Utilize built-in debugging tools or logs within the programming environment to trace and fix errors efficiently.
3. Maintaining Safety Protocols
Input integration should always prioritize safety, especially in environments where robots operate near humans or other machinery.
- Define Emergency Inputs: Program inputs that immediately halt the robot during emergencies (e.g., an emergency stop button or collision detection).
- Monitor Input Status Continuously: Ensure the robot regularly checks critical inputs to avoid unexpected malfunctions.
- Follow Industry Standards: Adhere to safety guidelines and protocols like ISO 10218-1/2 for industrial robot systems.
Use Cases
Inputs play a crucial role in making robot programs adaptive and responsive to real-world conditions. Here are two practical examples that highlight their importance:
1. Adding a Sensor to Stop the Robot in Emergencies
In industrial environments, safety is paramount. By integrating sensors like proximity or collision detectors, robots can stop immediately when they detect an obstacle or a human in their path.
- Example:
A robot in a manufacturing line is equipped with a proximity sensor. If the sensor detects an object in its predefined safe zone, it sends an input signal to the program, triggering the robot to stop to prevent a collision. This input-driven action reduces accidents and ensures workplace safety.
2. Using External Inputs for Collaborative Robotics
Collaborative robots (cobots) often work alongside humans, requiring seamless interaction through external inputs. These inputs can come from devices like cameras, force sensors, or user commands, enabling cobots to adapt to human workflows.
- Example:
A cobot assembling products uses a force sensor to determine the amount of pressure needed to tighten screws. If the input indicates excessive force, the robot adjusts its torque to avoid damage. Similarly, a human operator can issue voice commands to guide the cobot during complex tasks.
Hotel Jobs in Montreal Canada 2025
Conclusion
Integrating inputs into robot programs is a transformative approach that enhances their functionality, adaptability, and safety. From emergency stops triggered by sensors to collaborative workflows powered by external inputs, these use cases demonstrate how inputs empower robots to operate intelligently in dynamic environments.
By understanding and applying input integration techniques, industries can unlock the full potential of robotic automation, improving productivity and safety while reducing operational costs. For readers looking to venture into robot programming, exploring the use of inputs is an excellent way to begin enhancing automation capabilities. Dive into this exciting field to create smarter, more responsive robotic systems that meet the demands of today’s fast-paced industries.
FAQs
1. What are the different types of inputs in robotics?
Inputs in robotics can be classified into three main types:
- Sensor Inputs: Data from devices like proximity sensors, cameras, and temperature sensors that provide real-time information about the robot’s environment.
- User Commands: Instructions entered manually, such as voice commands or through control panels.
- External Device Inputs: Signals from external systems like Programmable Logic Controllers (PLCs) or IoT devices to synchronize tasks.
2. How do inputs improve robotic efficiency?
Inputs enable robots to adapt dynamically to changing conditions, reducing downtime and improving precision. Real-time feedback allows robots to make informed decisions, minimizing errors and optimizing workflows. For example, sensors can detect anomalies and trigger corrective actions, ensuring smooth and efficient operations.
3. Can inputs be modified after programming?
Yes, inputs can often be modified after programming. Most robotic programming environments allow users to update or adjust input configurations based on operational requirements. This flexibility ensures robots remain adaptable to new tasks, changes in the environment, or upgraded hardware.